Build your next killer app with Firebase
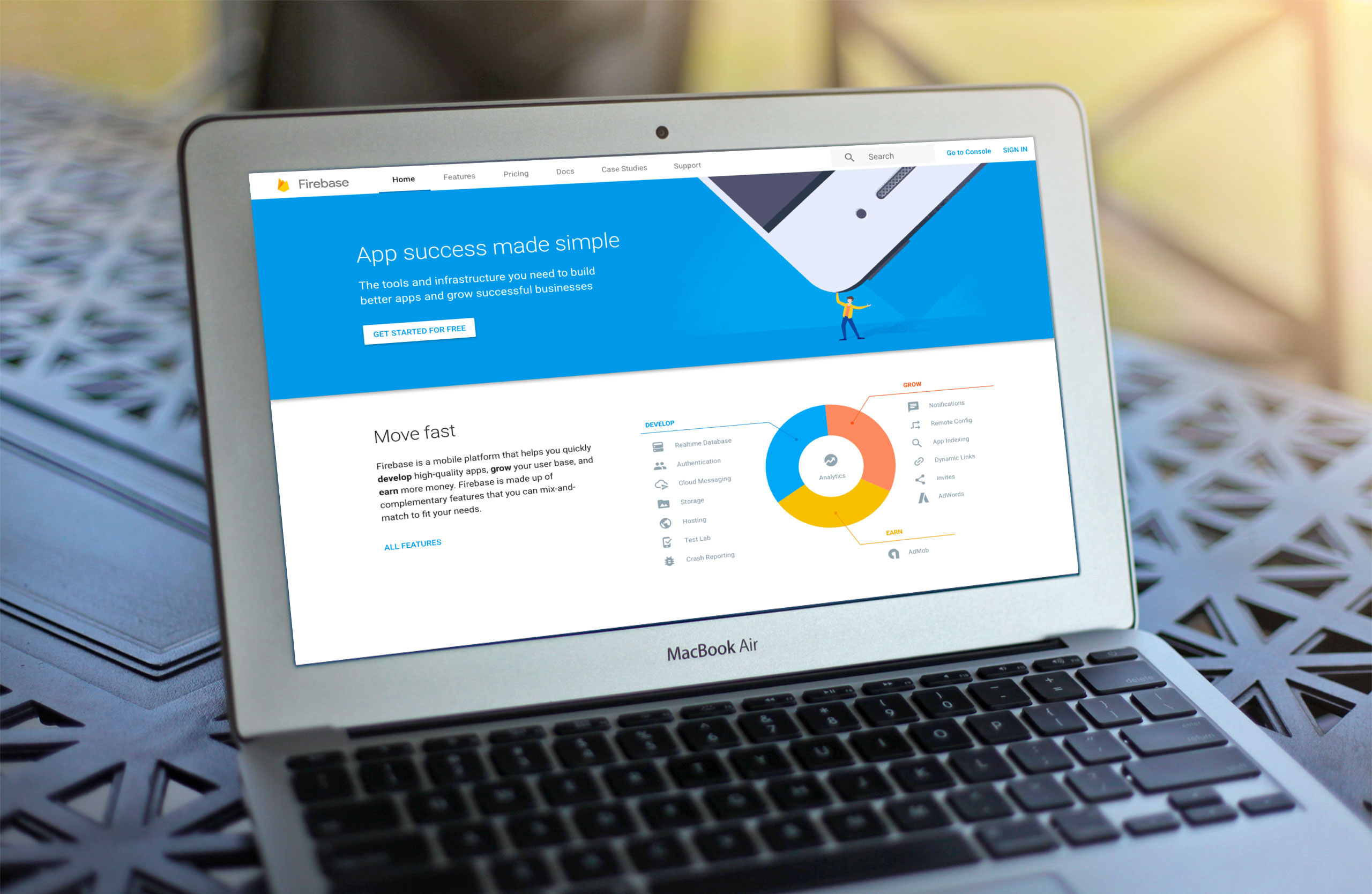
Always bootstrapping projects by myself, with friends, partners or clients worldwide, I’m used to be involved in discussing, designing and planning technical architectures. Today, I wanted to share some of these thoughts and studies about the tools available to help you build better applications, quicker. This post may be the first of a series “Build your next killer app with…“, describing scenarios and decisions taken during preparatory stages for various projects.
What is Firebase?
Some background: Firebase started as a realtime database in 2012, before being acquired by Google in 2014. It is now a set of tools, shipped as features such as (but not limited to) Storage, Hosting, Authentication, and Realtime Database, backed by Google infrastructure. Coming with libraries for both Android, iOS and web development, you’ll be able to work with it through multiple languages like Java, NodeJS, Javascript, and even C++. Documentation provides you with really useful guides for each feature, multiple code samples, and API references for all supported platforms.
Sounds good! Want more? It is really easy to bootstrap your application thanks to the Firebase Command Line Interface (CLI) Tools, that will help you initialize your project, host a local development environment, interact with your realtime database, and even deploy your code to the Firebase infrastructure. Sure, you can also use it in your continuous integration/deployment process, through GitLab CI for example, with the appropriate access token.
Typical scenario
Let’s say you have to build an application you’ve been thinking of, or your are in the process of building your Minimum Viable Product (MVP) expecting to raise funds or apply to a business incubator. You’ll initially have to think about:
- creating and hosting a database,
- managing users registration and authentication,
- storing some user provided/generated content like pictures or files,
- and hosting your application backend and frontend code.
These are four usual steps, which comes with a lot of research and thinking: describing features needed now (and later), designing, evaluating scalability, chosing providers and servers, ensuring compatibility and overall availability, integrating federated identity providers like Twitter, Facebook or Github…
And there, you can take a deep breath and know: Firebase has your back! Seriously.
Realtime Database
This is probably the main feature that generates traction, the first feature which will make you really interested in Firebase. The Realtime Database is a NoSQL, JSON, cloud hosted database, synchronized in realtime to every connected client, automatically. In other words, without you configuring or installing anything server side, without even thinking about AJAX or websockets, you simply initialize your SDK (iOS, Android, Web) client side: your application now gets live JSON data updates from your database, and vice versa.
Somewhere between “awesome” and “crazy”, here is how to enable that realtime database magic on your website with less than 10 lines of JavaScript code:
firebase.initializeApp({
apiKey: "apiKey",
authDomain: "projectId.firebaseapp.com",
databaseURL: "https://databaseName.firebaseio.com",
storageBucket: "bucket.appspot.com"
});
// Your application realtime database in a variable
var database = firebase.database();
Maybe you felt disappointed when reading “JSON” and “NoSQL”. This is where Firebase Realtime Database Rules gets in the game. Even if it’s JSON and NoSQL database, it comes with an integrated rules system, all written in JavaScript within a JSON object. It does NOT make your database relational, but adds some backend logic to enable you:
- ensuring both authentication (user must be logged-in to access data) AND authorization (logged-in user can be verified to be authorized to access data)
- applying data validation server side, before creating or updating data,
- and defining indexes to speed up querying as soon as your data grows.
These rules lives on Firebase servers, and are enforced automatically at all times. There is no way a user can read or write data without you authorizing him/her to do it through these rules. There is no way your data becomes inconsistent as you are always sure it’s been validated before being created or updated.
Have a look at the introduction video below, or head to Firebase Realtime Database guide to get more details about how it will help you to build an application coming with amazing features, in little to no time.
Users authentication
When it comes to authenticating users, one could say it’s easy as many frameworks comes with an appropriate set of tools like Laravel authentication and Passport (API Authentication). Sure, but you’ll have to spin-off a server, manage it’s PHP configuration and it’s database over time, ensuring it stays available at all time and grows seamlessly with your application, as authentication is critical to any app: you definitely not want your users to be denied access to your services just because your authentication platform failed or timed out!
Again, Firebase does the job. In addition to providing a realtime database, Firebase comes with an integrated and project-wide users database, authentication backend services, and SDK (iOS, Android, Web). You can have anonymous users, email/password based authentication, federated identity providers (as to now: Google, Facebook, Twitter, GitHub), or even integrate with an existing application hosting your users base already.
Storing user data
Backed by Google Cloud Storage, Firebase Storage enables you to both store and serve all of your user-generated content at any scale (up to petabyte). You can manage file uploads (pause, resume, cancel) and monitor progress from your frontend application. And it comes with key security features: as per the realtime database, you will be able to write rules to manage users authentication and authorization to read, and write files on your application storage. Using it on your website, after initializing your application in JavaScript with firebase.initializeApp
is as easy as:
// Create a reference to 'resume.pdf'
var userResume = firebase.storage().ref().child('resume.pdf');
var file = ... // use the Javascript Blob or File API
userResume.put(file).then(function() {
console.log('Your resume has been uploaded!');
});
Hosting your code
Firebase is all about cloud hosted APIs and services, available through SDK in your desired platform. If you happen to build a web frontend application to consume your database, you will need to serve your HTML/CSS/JS files, and Firebase Hosting will help you do it the smart way.
As simple as issuing firebase deploy
command, your static content is provisionned on highly efficient, SSD backed, worldwide CDN servers from Google. You can configure your own domain name, and it all comes with zero-configuration SSL support, even on your custom domain! Never worry again about buying an SSL certificate, or creating and renewing a Let’s Encrypt free certificate.
Awesome! What else?
Many other integrated services, like Analytics, Crash Reporting and AdMob monetization platform (for iOS and Android applications)… While it’s focused on, and initially targetting mobile applications, you’ll be able to leverage main Firebase features on your websites and web applications in no time with its JavaScript SDK.
Speaking about price, you’ll be amazed, too. Building your fully functionnal MVP or your next killer app with Firebase will cost you nothing at all (read $0), till your application grows, thanks to the free pricing plan, provided with (very) generous limits. A $25 monthly plan will boost your application limits. And when it’s not a question of price, but scalability and availability, you can reach the skies with basically unlimited services on a “pay as you go” basis, even giving you access to more of Google Cloud Platform. All without any reconfiguration, code changes, or servers provisionning.
What’s wrong?
Amazing, fabulous, awesome… Firebase is! But it also comes with some drawbacks:
- you’ll have to recreate your data validation rules on your frontend application, as you can’t export your database validation rules “as is”,
- architecturing your data may feel unusual to you if you’re coming from relational databases, and you’ll have to implement duplicates while thinking flat,
- depending on the application you have to build, backend code could be fully integrated in Firebase Realtime Database Rules, but as soon as you’ll need to implement other user stories, you may get to host more backend code on a server,
- and finally… you are tied to Google!
Now, what’s next? As you will hopefully be trying Firebase through the free plan for your new project, I may be picking alternatives and blog about it to help you build your next killer app, without these caveats.
Are you using Firebase, or planning to? Do you need help building your Minimum Viable Product?
I would love to get your feedback! Get in touch right now by email.
- Firebase: https://firebase.google.com/
- Guides: https://firebase.google.com/docs/web/setup
- Samples: https://firebase.google.com/docs/samples/
- Command Line Interface (CLI): https://github.com/firebase/firebase-tools
- Pricing: https://firebase.google.com/pricing/