Building an API without a server
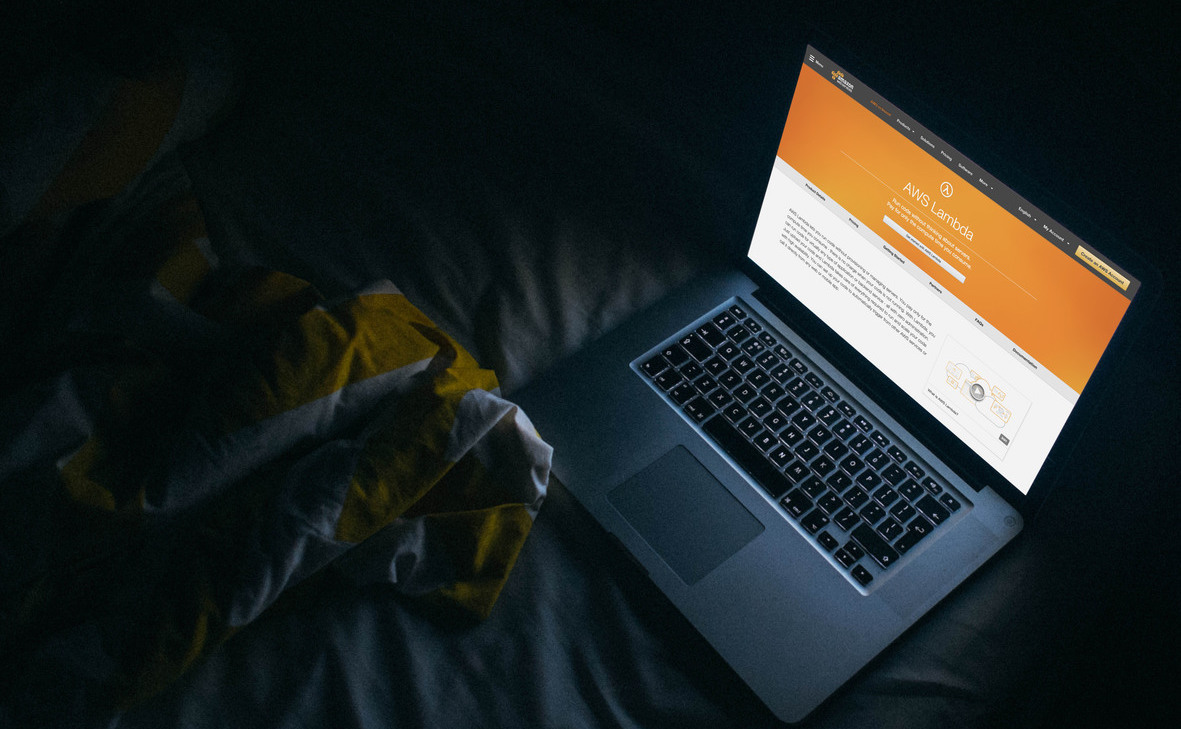
If you are used to traditional web development, in love with your top-notch coding framework, learning hard UNIX and Bash 101 to achieve the ultimate Apache or NGINX configuration on your newly deployed bare-metal/cloud/vps server offering pretty impressive capabilities for its affordable monthly charge, and don’t want to be shocked by the price it would cost you to reach unbeatable simplicity to deploy your next high availability API with nearly infinite scalability, you should definitely stop reading here.
Let’s get right to the point and leave no room for suspense. As of today here is the price of 1 million requests per month: free, each month, until you reach the next million requests on the same monthly period which will cost you $0.20 (for the 2nd million of requests, the first is still free). No kidding. Then, they charge you for the compute instance on a memory and time basis at the rate of $0.00001667 per GB memory per second. As an example, 100ms with 128MB memory allowed will cost $0.000000208. Most argue this is something painful with Amazon Web Services (AWS), having to use your calculator. I bet on you laughing about all this after your first month next to zero penny…
Now I got your attention, let’s do what we love to do most: building awesome stuff! Start by signing up for AWS. Pretty straightforward, yet highly secured as you will see it by yourself. When you get your account, and more important a new secured AWS Identity and Access Management (IAM) user, within your AWS Management Console use the Search services text box to get to the AWS Lambda console, in which you just have to click on Get Started Now.
Choose the microservice-http-endpoint
blueprint which will configure most of the details for you, pick a name and choose Open for security on the Configure triggers tab: you may completely remove your Lambda function after this demo, or play with higher security mechanism like traditional API keys or IAM for a full control over security management. Then, replace the code of your function with this simple example:
'use strict';
// this is what is called when the AWS Lambda Handler is 'index.handler'
exports.handler = (event, context, callback) => {
// you have full access to the request
console.log('Here is your request:', JSON.stringify(event, null, 2));
// with the callback function, you have full access to the response
const done = (err, res) => callback(null, {
statusCode: err ? '400' : '200',
body: err ? err.message : JSON.stringify(res),
headers: {
'Content-Type': 'application/json',
},
});
switch (event.httpMethod) {
case 'GET':
console.log('I love my job');
// sending a response
done(null, {'msg': 'GETing the world on a plate!'});
break;
default:
done(new Error(`Unsupported HTTP method "${event.httpMethod}"`));
}
};
Do not change the Handler for now: index.handler
means that AWS will call the exported handler
function from the index.js
file, which name is the default for your code when Node.js is chosen as the programming language. Here, the blueprint selected Node.js for you. Give the role a name and remove the policy template configured by the blueprint (we won’t use DynamoDB here). Then, stick to 128MB of memory with a 10s timeout (your function cannot execute longer, it will be killed after this timeout, remember you pay per compute time), and no custom VPC. Review and create your Lambda function.
When creation succeeds, hit the Test button. Let’s simulate an HTTP GET
request with this event:
{
"httpMethod": "GET"
}
Look at the execution results! You should have things like: Duration: 0.52 ms
and Billed Duration: 100 ms`. Remember the price for it, your tests are actually using computing instances and billed. Pay attention not to exceed 1 million… Amazon is offering a free tier, which to date is 12 month of free services (!!) from your sign-up date: that should be enough to get you started, and the free tier limits is quite high before being billed the normal (inexpensive) price.
With your test being green, I’m sure you can’t wait to test your API live, aren’t you? Easy! On your lambda function page, get on the Triggers tab. The blueprint selected earlier already configured an API Gateway for your Lambda function. An API Gateway gives you deep control over your public facing API: methods allowed, throttling, etc… For now, simply copy the fully qualified URL that has been attached to your Lambda function through the API Gateway, and test it live:
curl https://your.apigateway.endpoint.amazonaws.com/prod/LambdaMicroservice
Believe it or not: it was that easy. You’ve always been some clics away from your API being supercharged by AWS highly available infrastructure, without you ordering, installing, configuring and maintaining any server.
I've always been 60s away from my API being supercharged by @awscloud highly available services Tweet this
Read their docs and watch their presentation video: AWS Lambda is ready for one, or millions of requests per seconds. You will only pay for the requests your API received and the compute time given the memory you chose to allow, which is not a fixed price: less visitors and calls means cheaper bill, huge amount of visitors may be meaning fame and fortune to you… for some bucks on the AWS bill! This is just the beginning, dig in AWS Website and your AWS Management Console to discover your new super powers.
Great, but I love my console!
So do I! Let’s install AWS Command Line Interface (CLI). As usual, keeping your computer super-clean is as simple as starting your new sandbox in a throwable Docker container:
# Bash in a container with Python 3.X inside
docker run -it --rm python:3 /bin/bash
Never played with Python? You may love it. Funny fact: it is said to be the most widely used programming language in Alphabet companies. You will first have to wait a little for Docker images to download on your computer. When your sandbox playground is ready (ie within your Docker container):
pip install awscli
At this exact moment, staring at these downloading bars, dependencies installed and updated, I’m repeating myself this is what I love with Docker: never worrying about uninstalling.
This is what I love with #Docker: never worrying about uninstalling. Tweet this
As soon as it is installed, you will need to authenticate this AWS CLI. Create an access key from your IAM user, to be used right away:
aws configure
Verify you did well by listing your AWS Lambda functions attached to the account:
aws lambda list-functions
The world is all yours now: from the confort of your console, you have access to each and any service of the AWS ecosystem! What will you automate with it?
Want to know what I built from here? Need someone to help you building your next API on AWS?
I would love to get your feedback! Get in touch right now by email.
- Amazon Web Services (AWS): https://aws.amazon.com/
- AWS Free Tier: https://aws.amazon.com/free/
- AWS Lambda: https://aws.amazon.com/lambda/
- Getting Started: http://docs.aws.amazon.com/lambda/latest/dg/getting-started.html